3d Solar System With Opengl And Dev C++
Posted By admin On 12.12.20I am trying to make a solar system in OpenGL but the planet is not revolving around sun. That is revolving around some other axis. How to fix this? First, I am drawing a sun, Then, there is rotation, translation and again rotation of first planet. So it should revolve around the sun and rotate on it's own axis, but that's not happening. I am creating a solar system in OpenGL C in which the earth revolve around the sun. The only problem that i face now that i can't create stars above the window i tried everything but not helped Now i have deleted some extra code from here i hope it will work for you guys as those functions were not neccessary or making any change. Modelling the solar system in openGL closed Ask Question Asked 6. I'm looking to mode the solar system within c and openGL, and I was wondering if there was a cheap method that I could produce that would return an x,y,z vector that I could use to update the position of each planet every frame. Or other system in OpenGL I would. Solar system opengl free download. Pygame Pygame is a Free and Open Source python programming language library for making multimedia applicati. Manage a small solar system with - MPPT from victron - inverter - battery monitoring - logging. The Irrlicht Engine is an open source high performance realtime 3D engine written and usable in C. May 28, 2016 Small model solar system using OpenGL library. GitHub Gist: instantly share code, notes, and snippets.
Important!
To make application work, you need to download this res.tar.gz file :https://ufile.io/76lfk
then move it to cmake-build-debug folder and launch ./install.sh scriptORuntar file on your own and this should work too
This is because github didn't accept files as big.
Screenshots
Prerequisites
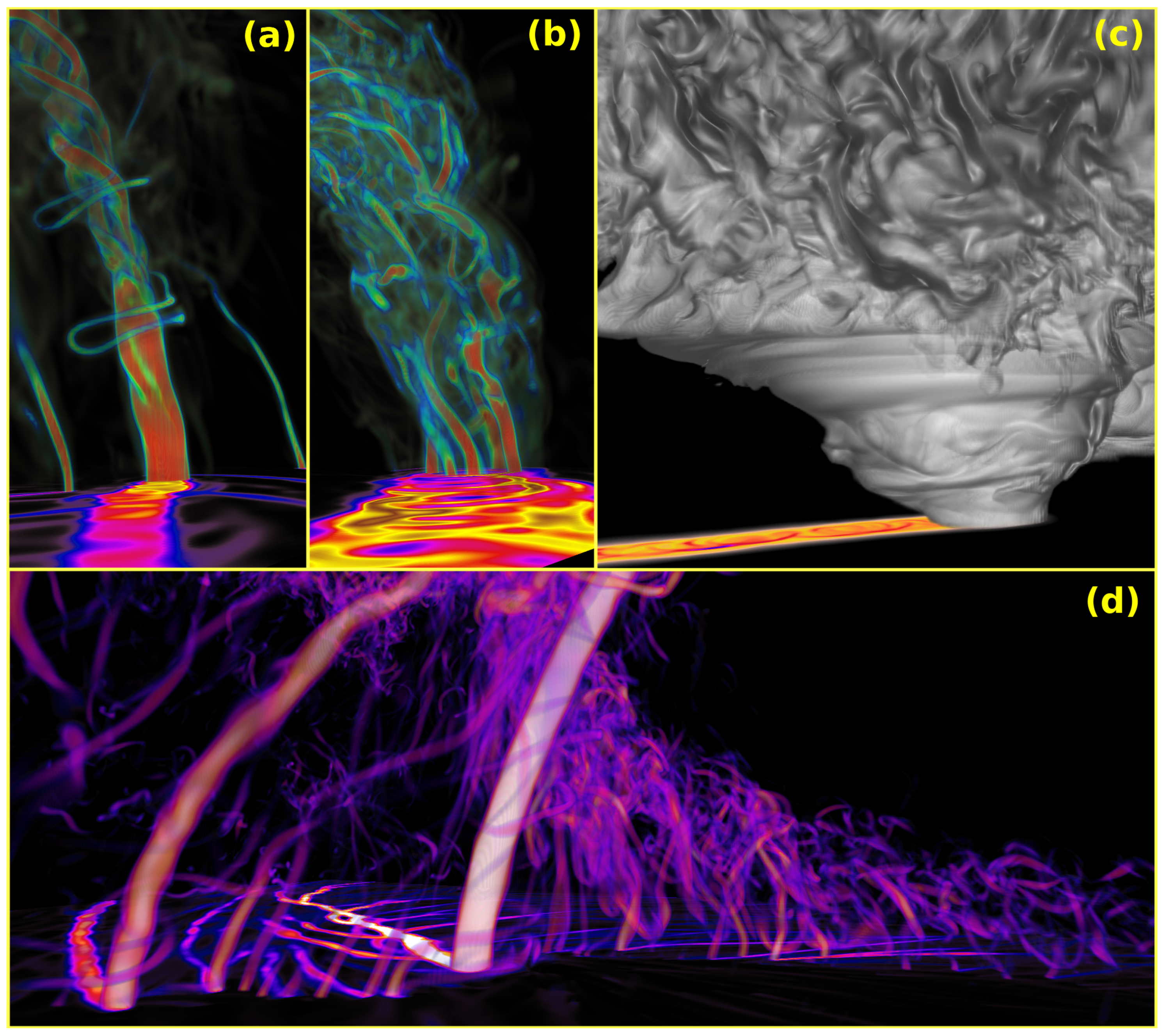
3d Solar System With Opengl And Dev C Download
- As said before, downloaded res.tar.gz
- Linux distro to launch binary (./cmake-build-debug/glSolarSystem)
[TO COMPILE CODE]
- GLEW library installed
- GLFW library installed
- glm library installed (single header library)
- OpenAL C++ implementation library installed
- ALUT library installed
About
This OpenGL Open Source project is one of my first OpenGL excercises,what I learned and implemented is loading obj files, textures, lighting, 3D audio.Made with CLion.
Compiling

Author
- Marcel Iwanicki - Initial work - Estebanan
License
This project is licensed under the MIT License - see the LICENSE.md file for details
Resources
Textures:https://www.solarsystemscope.com/textures/
Data:https://theplanets.org/distances-between-planets/http://planetfacts.org/orbital-speed-of-planets-in-order/https://en.wikibooks.org/wiki/GLSL_Programming/GLUT/Textured_Sphereshttps://en.wikipedia.org/wiki/Solar_rotation
Anti auto tune lyrics. References:https://www.khronos.org/https://www.youtube.com/user/ThinMatrixhttps://www.youtube.com/user/thebennyboxhttps://www.openal.org/documentation/OpenAL_Programmers_Guide.pdf
3d Solar System With Opengl And Dev C 4
/* |
* A simple inaccurate solar system animation. |
* |
* @author: itsGNUlinuxNOTjustLINUX |
* date: 5-28-2016 |
*/ |
#include<cmath> |
#ifdef __SUN__ |
#include<GLUT/glut.h> |
#else |
#include<GL/glut.h> |
#endif |
#include<stdlib.h> |
#include<cstdio> |
#include<string> |
/* GLUT callback Handlers */ |
staticvoidresize(int width, int height) |
{ |
constfloat ar = (float) width / (float) height; |
glViewport(0, 0, width, height); |
glMatrixMode(GL_PROJECTION); |
glLoadIdentity(); |
glFrustum(-ar, ar, -1.0, 1.0, 2.0, 100.0); |
glMatrixMode(GL_MODELVIEW); |
glLoadIdentity() ; |
} |
// Planet class that will hold all our planetary data |
classPlanet { |
public: |
std::string name = ''; |
double x = 0; |
double y = 0; |
double c1 = 0; |
double c2 = 0; |
double c3 = 0; |
double f = 0; |
double a = 0; |
double s1 = 0; |
Planet(std::string,double,double,double,double,double,double); |
voidglStuff(double t, double closeness); |
voidprintPlanet(); |
}; |
// Simple planet constructor |
Planet::Planet(std::string name='',double c1=0,double c2=0,double c3=0,double f=0,double a=0,double s1=0) { |
this->name = name; |
this->x = 0; |
this->y = 0; |
this->c1 = c1; |
this->c2 = c2; |
this->c3 = c3; |
this->f = f; |
this->a = a; |
this->s1 = s1; |
} |
// Function will compute new planet position |
voidPlanet::glStuff(double t, double closeness) { |
x = sin(t*f)*a*1.5; |
y = cos(t*f)*a; |
glColor3d(c1,c2,c3); |
glPushMatrix(); |
glTranslated(x,y,closeness); |
glRotated(50.0*t,0,0,1); |
glutSolidSphere(s1,20,20); |
glPopMatrix(); |
} |
staticdouble closeness = -15.0; |
Planet** planets; |
double moon_x; // moon x position |
double moon_y; // moon y position |
staticdouble t; // time |
staticvoiddisplay(void) // void |
{ |
t = glutGet(GLUT_ELAPSED_TIME) / 1000.0; |
glClear(GL_COLOR_BUFFER_BIT GL_DEPTH_BUFFER_BIT); |
for(int i = 0; i < 9; i++) { |
(*planets[i]).glStuff(t, closeness); |
} |
// moon orbit calculation |
moon_x = -sin(t)*0.5+ planets[3]->x; |
moon_y = cos(t)*0.5+ planets[3]->y; |
// 'Moon' |
glColor3d(0.7,0.7,0.7); |
glPushMatrix(); |
glTranslated(moon_x , moon_y , closeness); |
glRotated(60,1,0,0); |
glRotated(50.0*t,0,0,1); |
glutSolidSphere(0.1,20,20); |
glPopMatrix(); |
glutSwapBuffers(); |
} |
staticvoidkey(unsignedchar key, int x, int y) |
{ |
switch (key) |
{ |
case27 : |
case'q': |
exit(0); |
break; |
case'+': |
if (closeness < -4.0) { |
closeness += 0.5; |
} |
break; |
case'-': |
closeness -= 0.5; |
break; |
} |
glutPostRedisplay(); |
} |
staticvoididle(void) |
{ |
glutPostRedisplay(); |
} |
const GLfloat light_ambient[] = { 0.0f, 0.0f, 0.0f, 1.0f }; |
const GLfloat light_diffuse[] = { 1.0f, 1.0f, 1.0f, 1.0f }; |
const GLfloat light_specular[] = { 1.0f, 1.0f, 1.0f, 1.0f }; |
const GLfloat light_position[] = { -20.0f, 10.0f, 0.0f, 0.0f }; |
const GLfloat mat_ambient[] = { 0.7f, 0.7f, 0.7f, 1.0f }; |
const GLfloat mat_diffuse[] = { 0.8f, 0.8f, 0.8f, 1.0f }; |
const GLfloat mat_specular[] = { 1.0f, 1.0f, 1.0f, 1.0f }; |
const GLfloat high_shininess[] = { 50.0f }; |
intmain(int argc, char *argv[]) |
{ |
// Although the sun is not a planet it is |
// convenient to keep in our array of planets |
planets = new Planet*[9]; |
Planet q('sun', 1.0, 0.6, 0.0, 0.0, 0.0, 1.5); planets[0] = &q; |
Planet a('mercury', 0.5, 0.4, 0.4, 1.0, 2.0, 0.15); planets[1] = &a; |
Planet s('venus', 0.6, 0.6, 0.2, 0.5, 3.0, 0.18); planets[2] = &s; |
Planet d('earth', 0.0, 0.0, 0.7, 0.2, 4.0, 0.2); planets[3] = &d; |
Planet f('mars', 1.0, 0.2, 0.0, 0.15, 5.5, 0.15); planets[4] = &f; |
Planet z('jupiter', 0.8, 0.6, 0.3, 0.12, 7.0, 0.5); planets[5] = &z; |
Planet x('saturn', 0.9, 0.8, 0.3, 0.1, 10.0, 0.15); planets[6] = &x; |
Planet c('uranus', 0.4, 0.4, 1.0, 0.08, 13.0, 0.35); planets[7] = &c; |
Planet v('neptune', 0.0, 0.0, 0.5, 0.05, 17.0, 0.35); planets[8] = &v; |
glutInit(&argc, argv); |
glutInitWindowSize(1100,600); |
glutInitWindowPosition(100,20); |
glutInitDisplayMode(GLUT_RGB GLUT_DOUBLE GLUT_DEPTH); |
glutCreateWindow('Solar System'); |
glutReshapeFunc(resize); |
glutDisplayFunc(display); |
glutKeyboardFunc(key); |
glutIdleFunc(idle); |
glClearColor(0,0,0,0); |
glEnable(GL_CULL_FACE); |
glCullFace(GL_BACK); |
glEnable(GL_DEPTH_TEST); |
glDepthFunc(GL_LESS); |
glEnable(GL_LIGHT0); |
glEnable(GL_NORMALIZE); |
glEnable(GL_COLOR_MATERIAL); |
glEnable(GL_LIGHTING); |
glLightfv(GL_LIGHT0, GL_AMBIENT, light_ambient); |
glLightfv(GL_LIGHT0, GL_DIFFUSE, light_diffuse); |
glLightfv(GL_LIGHT0, GL_SPECULAR, light_specular); |
glLightfv(GL_LIGHT0, GL_POSITION, light_position); |
glMaterialfv(GL_FRONT, GL_AMBIENT, mat_ambient); |
glMaterialfv(GL_FRONT, GL_DIFFUSE, mat_diffuse); |
glMaterialfv(GL_FRONT, GL_SPECULAR, mat_specular); |
glMaterialfv(GL_FRONT, GL_SHININESS, high_shininess); |
glutMainLoop(); |
return EXIT_SUCCESS; |
} |